Introduction
In this blog article I am going to show you, how I personally develop my .NET MAUI apps. Just a quick note before we begin: this is my personal approach to .NET MAUI development. I’m not claiming it’s the only way or even the best way, but it’s what works for me. So, if you’re curious to see how I build, structure, and deploy my apps, read on.
I splitted the article in 5 sections. First we take a look at the common tools that I use to develop my apps, then I will show and explain to you how I typically structure my projects. After that we look at my way of handling the UI, then I give a quick overview over my backend choices and finally I give to you the most valuable nuget packages, that I use for speeding up the development process in every app of mine.
Lets get started 🙂
Tools
This is a quick one since you really don’t need much to develop apps besides your brain, a laptop and an IDE and preferably an internet connection. So lets take a look.
- Laptop: I use my 2 year old ThinkPad E14 Gen 4 with a Ryzen 7 (around 1000$ at the time) connected to 2 external monitors to write my code.Â
- IDE: My IDE of choice is Visual Studio by Microsoft, which has a perfect integration for MAUI and .NET in general. I also tried VS Code for a while but eventually switched back to Visual Studio as I’m feeling much more comfortable with it.
- AI Helpers: I personally use GitHub Copilot, which I get for free through my student program and besides that I typically use Claude AI and ChatGPT depending on where I already reached the free limitÂ
Project Structure
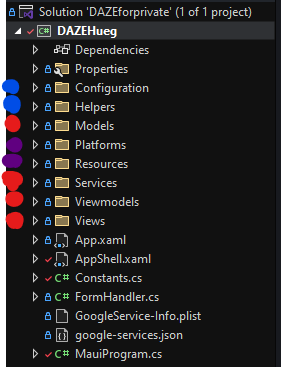
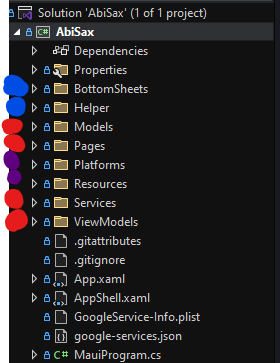
As you can see in those images, which showcase the project structure of my 2 latest apps I typically have the same structure in every project. This is what works for me and now I will explain that in further detail.
First all folders marked violet are preexisting for every new MAUI project. The blue folders are additional things i don’t need every time. Typically the Helper folder is for storing Converters and stuff like. the others are specific to the apps. One could argue that BottomSheets should be part of the views and he would be right. I can’t actually remember why I separated that.
Where I figured out the best way for me is with the red marked folders. Much of that has to do with the architectural pattern that I use, which is called MVVM (Model–view–viewmodel). This explains the 3 main red folders of Models, Views/Pages and ViewModels. The addition I made, which I think is not that common, is the Services folder. But what do those terms even mean in the first place? General definition coming:
MVVM (Model-View-ViewModel)
MVVM is a design pattern used primarily for building user interfaces that separates the concerns of data (Model), user interface (View), and the logic that connects them (ViewModel). This separation offers several benefits like improved testability, maintainability, and code reusability.
Components
Model: Represents the data and business logic of the application. It is responsible for fetching, storing, and manipulating data. It has no direct knowledge of the View or ViewModel.
View: The user interface that displays data from the ViewModel and allows the user to interact with the application. It is typically implemented using XAML or HTML and has no direct knowledge of the Model.
ViewModel: Acts as an intermediary between the Model and the View. It prepares data from the Model in a way that is easily consumable by the View. It also handles user interactions and updates the Model accordingly. It has no direct knowledge of the View.
How it works together
The View observes the ViewModel for changes, and the ViewModel observes the Model. When the Model changes, the ViewModel is updated, which in turn updates the View. User interactions in the View trigger commands in the ViewModel, which then updates the Model. This ensures that the Model, View, and ViewModel remain loosely coupled, making the application easier to develop, test, and maintain.
Example
Imagine a simple application that displays a list of customers.
Model: Would be responsible for fetching the customer data from a database or API.
View: Would display the list of customers in a user-friendly way, perhaps using a list or grid.
ViewModel: Would retrieve the customer data from the Model, format it for display (e.g., sorting, filtering), and expose it to the View.
This separation of concerns allows developers to work on different parts of the application independently, improving productivity and reducing complexity.
Now I’ll explain how I use this for developing my apps and which changes/additions I made. Concerning Views and ViewModels I just use them as in the definition explained. Her I have no further things to add.
Concerning the Models tho I have quite a different approach. I personally use Models as pure definitions of the Data they hold meaning they are quite stupid and just hold data instead of for example fetching or manipulating it. Those are the tasks that the Services do in my applications. Below I have two pictures showing exactly that. For the model you can see that it clearly only defines the structure of the data without doing anything. The service on the other hand is able to talk to the appstore api and use that model to purchase a subscription for example.
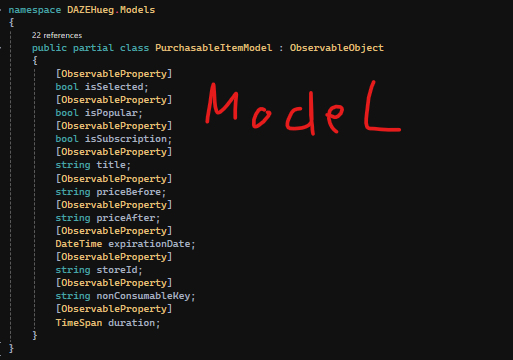
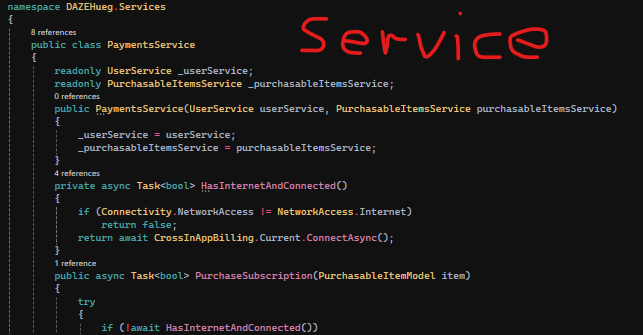
UI
My approach to UI design is relatively basic. I start by defining the color palette for my app and storing it in a dictionary. I tend to stick with the standard MAUI styles and simply modify the colors to match my design. I realize there’s room for improvement here, and I’m always looking for ways to enhance my UI skills. To help streamline the process, I utilize these packages:
- Uranium UI: https://enisn-projects.io/docs/en/uranium/latest/ –Â Modern, extensible, customizable, and easy to use UI framework for .NET MAUI. Uranium is a Free & Open-Source UI Kit for .NET MAUI. It provides a set of controls and utilities to build modern applications. It is built on top of the .NET MAUI infrastructure and provides a set of controls and layouts to build modern UIs. Really love it!
- Freaky Controls: https://github.com/FreakyAli/Maui.FreakyControls – Maui.FreakyControls is a UiKit for Maui, The repository includes a variety of controls that you can use to create unique and visually stunning user interfaces for your Maui applications. Each control is highly customizable and comes with a set of parameters that allow you to adjust them to your specific needs. – Not in every project and mostly for really specific things, but really enjoyable using
- BottomSheets:Â https://github.com/the49ltd/The49.Maui.BottomSheet
- Popups:Â https://github.com/LuckyDucko/Mopups
- Icons:Â https://github.com/AathifMahir/MauiIcons
Backend Choices
My backend philosophy is all about efficiency and ease of use. I prefer to avoid the complexities of managing servers and scaling infrastructure. That’s why I gravitate towards Backend as a Service (BaaS) solutions like Supabase and Firebase.
Here’s a breakdown of my thoughts on each:
- Firebase: A great choice for getting started, especially if you’re not anticipating a huge influx of users. It’s incredibly user-friendly and offers a generous free tier.
- Supabase: When you need a robust and scalable backend, Supabase is an excellent option. It integrates seamlessly with MAUI and provides a smooth setup experience. Here I also plan a tutorial series on my YouTube channel, so click the button on the right top of your screen 🙂
In some cases, I find it beneficial to utilize both platforms. I might use Firebase for features like Google Analytics, while relying on Supabase for its powerful database capabilities.
Most valuable nuget packages
Finally, let’s take a look at a list of NuGet packages that I incorporate into every app I build:
- CommunityToolkit.Maui – for a easier time developing in general https://learn.microsoft.com/en-us/dotnet/communitytoolkit/maui/get-started?tabs=CommunityToolkitMaui
- CommunityToolkit.Mvvm – for less boilerplate regarding UI changes https://learn.microsoft.com/en-us/dotnet/communitytoolkit/mvvm/
- Plugin.InAppBilling – for monetization https://jamesmontemagno.github.io/InAppBillingPlugin/GettingStarted.html
- Plugin.Maui.AppRating – for app rating https://github.com/FabriBertani/Plugin.Maui.AppRating
- Sentry.Maui – for crash reporting https://docs.sentry.io/platforms/dotnet/guides/maui/
- sqlite-net-pcl – for local storage https://learn.microsoft.com/en-us/dotnet/maui/data-cloud/database-sqlite?view=net-maui-8.0
- and the UI packages named in the UI part